Data Grid
JavaScript data grid that consumes JSON data and can handle large data sets. Seamlessly integrated with JSCharting, for many usage cases, there is no need for a separate JavaScript grid library.
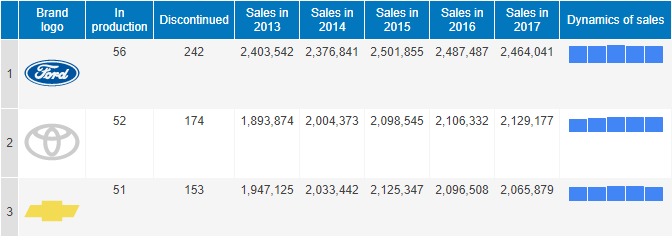
Introduction
JSCGrid is a javascript data grid component that can work as a stand-alone (no jquery or other frameworks required) HTML5 data grid that consumes JSON data. It can also be emitted by a chart to show the chart datatables in a grid format targeting a specific div on a page or as an alternative chart view. The chart can query server-side data and update the client-side html tables as needed.
The javascript grid supports token values similar to the chart labels making it easy to create columns with expressions and formatting. Microcharts, icons, and other features can also be added easily to the grid using the same text syntax as within chart labels.
A CSS file loads automatically but can be disabled and a custom CSS file based on the original can be used.
Grid is thenable
A grid requires an additional module to download. It can be included in the page script tag or will download on first use. Functions that return a grid, including the grid constructor will always return a thenable object. This means a .then() can follow the grid function to use the grid after it is created.
var grid;
JSC.Grid("gridDiv", {
/*Grid Options*/
}).then(function(g) {
grid = g;
});
If the datagrid.js module is included in the page script tag, the grid constructor will return a grid object. However, the grid object is still thenable.
<script src="./JSC/modules/datagrid.js"></script>
var grid = new JSC.Grid("gridDiv", {
/*Grid Options*/
});
Chart Based Grid
A chart offers a toGrid() function that emits the chart data to a grid inside the specified html div element.
var grid,
chart = new JSC.Chart(
"chartDiv",
{
/*Chart Options*/
},
function(c) {
c.toGrid("gridDiv").then(function(g) {
grid = g;
});
}
);
Charts can offer the alternative grid view by setting the chart based grid_enabled option to true.
var chart = new JSC.Chart("chartDiv", {
grid: { enabled: true }
/*Chart Options*/
});
Options are not required to generate a grid from a chart. The grid will automatically determine the data values, and formatting necessary based on chart options but everything can be customized by passing new options to the grid. Each chart data point is represented in the grid as a dataRow.
Chart bound grid column definitions
Column definitions can be defined to overwrite the default columns determined automatically with a high-performance microchart. An example:
var myGrid;
chart1.toGrid('gridDiv', {
columns:[{
header:'Series',
value:'%seriesName',
},{
header:'X Value',
value:'%xValue',
}, {
header:'% of Series',
value:'%percentOfSeries'
}, {
header:'Y Value Microchart',
// Microchart with tooltip
value:'<chart type=bar data=%yValue tooltip=%yValue max=200>'
},{
header:'Calculation',
// Expression with currency formatting
value:'{%yValue*5.23:c}}'
}
}]
}).then(function(grid){
myGrid=grid;
})
Refreshing chart based grid when chart data changes
When the following code runs, the grid will refresh values and apply any specified options.
myGrid.options({})
Stand-Alone DataGrid Data Binding
Array Data Source
Using an array of arrays as a data source.
See the Pen Docs - Array Data Source by Arthur Puszynski (@jsblog) on CodePen.
Array Data Source with Custom Columns
Array data columns can be referenced inside column value strings using tokens as shown below. This example skips some columns and adds others that use the hidden data in calculations.
var grid,
data = [
["Product", "Units Sold", "Revenue"],
["Hammer", 152, 1518.48],
["Saw", 1355, 27086.45],
["Nail Gun", 3355, 201266.45]
];
JSC.Grid("gridDiv", {
data: data.slice(1),
columns: [
{ header: "Product", value: "%0" },
{ header: "Units Sold", value: "%1", align: "right" },
{ header: "Revenue", value: "{%2:c}", align: "right" },
{ header: "Unit Price", value: "{%2/%1:c}", align: "right" }
]
}).then(function(g) {
grid = g;
});
Array of Objects Data Source
An array of objects can be used as a data source with similar token syntax to add calculation columns. A function can also be used to define column values to provide more complex calculation capabilities.
Data Grid Deep Sample Stand-alone data grid with nested JSON values.
JSC.csv2Json()
A CSV file content can be loaded into a grid very easily with a single line of code such as:
JSC.Grid("gridDiv", JSC.csv2Json(csvString)).then(function(grid) {});
The code above will parse CSV text, detect headers and data types, and render the grid with the CSV data inside the gridDiv element.
fetch(), CSV, and JSON Tutorial Loading CSV files
Usage
Column and Summary Definitions
Default Column
The grid defaultColumn option can be set to specify default column settings to avoid setting the same values for each column definition.
grid.options({
defaultColumn: { width: "150px" }
});
As seen in the above samples, each column can define the data it displays, headers, and other options. Column values can be customized to refer to any values in a given data row.
Summary Row
A row that summarized the data of a given column can be defined as well.
Each data column can be summarized with a calculation at the end. The grid summaryRow option can be defined with an array of values for each column, or an object with property names matching the source data properties. An array of either one can be used to define multiple summary rows.
grid.options({
summaryRow: ["Sum", "", "%sum"]
})
Grid Summary Row Sample A summary row defined for a data grid connected to a chart.
Tokens
Chart Based
The same tokens used in charts work grid cell values. See the Token Reference Tutorial.
Array/Object based
When data rows are arrays, tokens such as '%1' can be used, and they refer to the value at the data row array index. Object data rows use a similar syntax such as '%property' or nested object properties such as '%name.first'.
Data Grid Deep Sample Stand-alone data grid with nested JSON values.
File Export
The grid exportFile option enables a button that downloads the data shown in the grid as a CSV file.
CSS Styling
The grid component automatically downloads the datagrid.css file found in the JSC/resources/ folder. To modify the default grid styling, an additional css file can be defined and referenced in the html <head>. The default datagrid styles will load first.
To omit loading default styles, the grid cssFile property can be set to an empty string. Or the path of an alternative css file can be used with this property to load it automatically.
It may be easiest to make a copy of the default css file to modify and set the cssFile property to point to this new file. Or if the modified css file is merged with other website styles, setting the cssFile property to an empty string.
Microcharts & Icons
Microcharts can be inserted into grids for any cell. The same '<chart>' syntax can be used in column definition values as in chart labels. In addition, the '<icon>' syntax can be added to column values to insert svg icons into the grid.
Grid Custom Icons Sample Demonstrate using SVG images in data grids.